jsp展示数据
<%@page import="com.landing.bean.Product"%>
<%@page import="java.util.List"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<table border="1" cellpadding="10" cellspacing="0">
<tr>
<th>Id</th>
<th>Name</th>
<th>Price</th>
<th>Location</th>
</tr>
<%
List<Product> products = (List<Product>)request.getAttribute("products");
for(Product product : products) {
%>
<tr>
<td><%= product.getId() %> </td>
<td><%= product.getName() %> </td>
<td><%= product.getPrice() %> </td>
<td><%= product.getLocation() %> </td>
<%
}
%>
</tr>
</table>
</body>
</html>
通过Servlet获取数据源查询数据库
package com.landing.bean;
import java.io.IOException;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.Hashtable;
import java.util.List;
import javax.naming.Context;
import javax.naming.InitialContext;
import javax.naming.NamingException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.sql.DataSource;
public class ProductServlet extends HttpServlet{
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
System.out.println(getAllProducts());
req.setAttribute("products", getAllProducts());
req.getRequestDispatcher("/products.jsp").forward(req, resp);
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req, resp);
}
public List<Product> getAllProducts() {
List<Product> products = new ArrayList<Product>();
Hashtable env=new Hashtable();
env.put(Context.INITIAL_CONTEXT_FACTORY,"weblogic.jndi.WLInitialContextFactory");
env.put(Context.PROVIDER_URL, "t3://localhost:7001");
Context ctx = null;
DataSource ds = null;
Connection conn = null;
Statement statement = null;
ResultSet rs = null;
try {
ctx = new InitialContext(env);
ds = (DataSource) ctx.lookup("jdbc");
conn = ds.getConnection();
String sql = "select * from product";
statement = conn.createStatement();
rs = statement.executeQuery(sql);
while(rs.next()) {
String id = rs.getString("id");
String name = rs.getString("name");
String price = rs.getString("price");
String location = rs.getString("location");
Product product = new Product(id,name,price,location);
products.add(product);
}
} catch (NamingException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally{
if(rs != null) {
try {
rs.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
if(statement != null) {
try {
statement.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
if(conn != null) {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
if(ctx != null) {
try {
ctx.close();
} catch (NamingException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
return products;
}
}
Product 对应数据库表
package com.landing.bean;
public class Product {
private String id;
private String name;
private String price;
private String location;
public Product(String id, String name, String price, String location) {
super();
this.id = id;
this.name = name;
this.price = price;
this.location = location;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPrice() {
return price;
}
public void setPrice(String price) {
this.price = price;
}
public String getLocation() {
return location;
}
public void setLocation(String location) {
this.location = location;
}
}
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://xmlns.jcp.org/xml/ns/javaee" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" id="WebApp_ID" version="3.1">
<display-name>weblogic_demo</display-name>
<servlet>
<servlet-name>productList</servlet-name>
<servlet-class>com.landing.bean.ProductServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>productList</servlet-name>
<url-pattern>/productList</url-pattern>
</servlet-mapping>
</web-app>
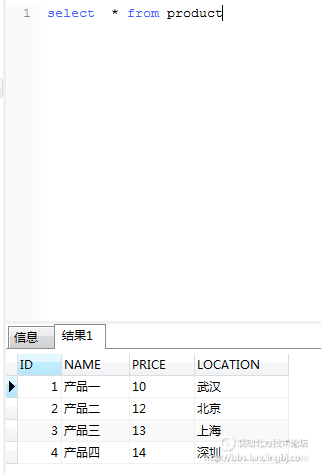
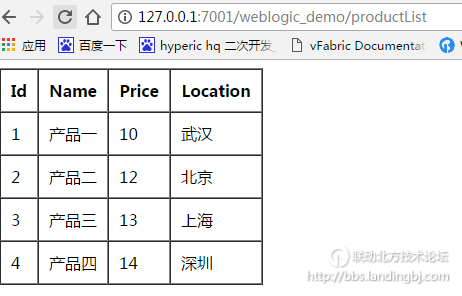