对于一个初学者来说,使用Dom解析和SAX解析xml多少有点复杂和难度,而dom4J解析是目前xml解析里面最优秀的一种方式,所以我们就看一看dom4j解析的例子。
首先,使用dom4j解析xml必须要有dom4j的jar包:dom4j-1.6.1.jar
接下来我们写一个简单的xml文件,用于Java解析,文件代码如下:
<?xml version="1.0" encoding="UTF-8"?>
<students name="Tom">
<hello name="jack">hello Text1</hello>
<hello name="jack2">hello Text2</hello>
<hello name="jack3">hello Text3</hello>
<world name="paul">world text1</world>
<world name="paul2">world text2</world>
<world >world text3</world>
</students>
新建一个Java类(dom4jTest2)解析如上xml文档。
Java代码如下:
import java.io.File;
import java.util.Iterator;
import java.util.List;
import org.dom4j.Document;
import org.dom4j.Element;
import org.dom4j.io.SAXReader;
public class Dom4JTest2 {
public static void main(String[] args) throws Exception{
SAXReader saxreader=new SAXReader();
Document document=saxreader.read(new File("students.xml"));
//获取根元素
Element root=document.getRootElement();
System.out.println("根元素Root:"+root.getName());
//获取所有子元素
List<Element> childlist=root.elements();
System.out.println("子元素共计"+childlist.size()+" 个");
//根据名称获取子元素
List<Element> childnamelist=root.elements("hello");
System.out.println("hello子元素共有:"+childnamelist.size()+" 个");
//获取指定名称子元素的第一个子元素
Element firstchildElement=childnamelist.get(0);
Element secondchildElement=childnamelist.get(1);
//输出其属性
System.out.println("第一个子元素属性"+firstchildElement.attributeValue("name")+" "+firstchildElement.getStringValue());
System.out.println("第二个子元素属性"+secondchildElement.attributeValue("name")+" "+secondchildElement.getStringValue());
System.out.println("迭代输出---------------------");
for(Iterator<Element> it=root.elementIterator();it.hasNext();){
Element e=it.next();
System.out.println(e.attributeValue("name"));
}
}
}
运行结果:
控制台输出如下:
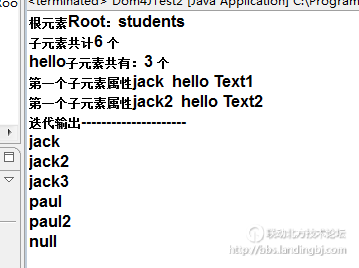
这样一个简单的dom4j的示例就完成了。
后来在网上找了一下关于Java解析XML的方法,发现这个博客里面写的很详细也很全面,博客地址如下:
http://blog.csdn.net/smcwwh/article/details/7183869
http://www.jb51.net/article/42323.htm